自定义ele树形控件样式
# 自定义ele树形控件样式
# 通过 scoped-slot
实现自定义树形控件
效果图:
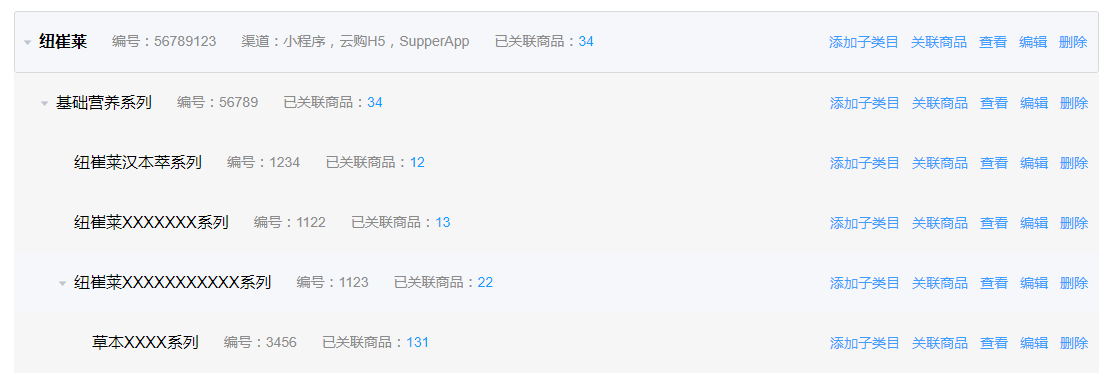
# 实现
html代码:
<el-tree
:key="index"
:data="data" // 树形控件数据源
node-key="id" // 每个树节点对应的唯一标识符
draggable // 是否可以拖拽
>
// 插槽,放自定义节点内容
// 此处的class:custom-tree-node和slot-scope均是固定写法
<div
slot-scope="{ node, data }" // 通过slot-scope 绑定数据
class="custom-tree-node tree-category"
>
<div class="tree-category-title">
<div
:class="{ firstTitle: node.level === 1 }"
class="title"
>
//label标题
{{ data.title }}
</div>
<div class="text">
编号:{{ data.number }}
</div>
<div
v-if="node.level === 1"
class="text"
>
渠道:{{ data.channel }}
</div>
<div class="text">
已关联商品:<span>{{ data.associated }}</span>
</div>
</div>
<div class="tree-category-button">
<el-button type="text">
添加子类目
</el-button>
<el-button type="text">
关联商品
</el-button>
<el-button type="text">
查看
</el-button>
<el-button type="text">
编辑
</el-button>
<el-button type="text">
删除
</el-button>
</div>
</div>
</el-tree>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
css代码:
.el-tree {
margin: 10px 0;
.el-tree-node {
.el-tree-node__content {
border: 1px solid #d9d9d9;
border-radius: 3px;
padding: 10px;
height: auto;
.tree-category {
display: flex;
justify-content: space-between;
align-items: center;
width: 100%;
.tree-category-title {
display: flex;
justify-content: space-between;
.firstTitle {
font-weight: bold;
}
.title {
font-size: 16px;
color: #000;
margin-right: 25px;
}
.text {
color: #8b8b8b;
font-size: 14px;
font-weight: 400;
margin-right: 25px;
span {
color: #0c9dfe;
}
}
}
}
}
// 因为 tree 是递归组件,所以给一级树设置边框时后续所有级都会带有边框
.el-tree-node__children {
background-color: #f6f6f6;
.el-tree-node__content {
border: none;
}
}
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
js代码:
data() {
return{
// 树形控件的数据源,为数组。
data: [
{
id: "",
title: "",
child: [
{
id:"",
title: "",
child: []
}
]
}
],
// 树形控件配置项,为对象,label代表节点的title标签,children代表节点的子树(孩子)
defaultProps: {
label: 'title',
children: 'child'
},
// 默认展开的数组
expandKeys: [],
}
}
// 在树形结构渲染到页面之前,需要后台先获取树形控件的数据源
// 在mounted()中调用获取树形控件数据源的函数
mounted() {
this.getTreeData();
},
// 上方法
methods: {
// 获取树形结构的数据源
getTreeData() {
// 调用api接口,获取数据库数据库数据
ConfigApi.getCategoryTree().then(res => {
if (res.code === 200) {
this.treeData = res.result;
// 设置默认选中和默认展开的节点
setTimeout(() => {
// 如果树形控件的数据源,则将其第一个节点设置为当前选中的节点 及 当前展开的节点
if (this.treeData.length > 0 ) {
this.setCurrentKey(this.treeData[0].id);
this.expandKeys = [this.treeData[0].id];
}
}, 0);
}
});
},
// setCurrentKey为element官网自带的通过key设置当前某个节点选中的状态,使用此方法必须设置node-key属性
// 注意:(key)待被选中的节点key, 若为null则取消当前高亮的节点
setCurrentKey(key) {
// 如果存在当前传入的参数key,则为当前树形控件设置选中的节点
if (key) {
this.$refs.tree.setCurrentKey(key);
// 当前节点发生变化时,触发
this.currentNode(
null,
// 先获取当前被选中节点的key,再根据选中的节点key拿到组件中的node
this.$refs.tree.getNode(this.$refs.tree.getCurrentKey())
);
}
},
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
在GitHub上编辑 (opens new window)
上次更新: 2/23/2022, 5:36:03 PM