二维码插件
# 二维码插件
官网:node-qrcode (opens new window)
# 安装
npm install --save qrcode
# 使用
全局引入:
// main.js
import qrcode from 'qrcode'
Vue.prototype.$qrcode = qrcode
2
3
# 通过canvas绘制
使用方式:
toCanvas(canvasElement, text, [options], [cb(error)])
toCanvas(text, [options], [cb(error, canvas)])
将二维码绘制到canvas上,如果canvasElement
省略则返回新的画布。
属性:
canvasElement
类型:
DOMElement
规定在哪个画布上绘制
text
类型:String|Array
规定二维码的内容,可以是文字描述,可以是url链接等。
options
其它设置参考选项
cb
回调函数
例子:
<canvas ref="qrcode"></canvas>
onCanvas(){
this.$qrcode.toCanvas(
this.$refs.qrcode,
text,
function (err) {
if (err) throw err;
console.log('success!');
}
);
}
2
3
4
5
6
7
8
9
10
# 通过img绘制
使用方式:
toDataURL(text, [options], [cb(error, url)])
toDataURL(canvasElement, text, [options], [cb(error, url)])
返回包含 QR 码图像表示的数据 URI。 目前仅适用于image/png类型。
属性:
canvasElement
类型:
DOMElement
规定在哪个画布上绘制
text
类型:String|Array
规定二维码的内容,可以是文字描述,可以是url链接等。
options
type
类型:
String
默认值:image/png
数据 URI 格式。 可能的值为:
image/png
,image/jpeg
,image/webp
。rendererOpts.quality
类型:
Number
默认值:0.92
如果请求的类型是
image/jpeg
或image/webp
,则介于0
和之间的数字1
指示图像质量。`
其它设置参考选项
cb
回调函数
例子:
<img ref="qrCodeImg" />
...
options: {
errorCorrectionLevel: "H",
type: "image/png",
quality: 0.3,
margin: 1,
color: {
dark:"#010599FF",
light:"#ffffffff"
},
},
...
onImg(){
this.$qrcode.toDataURL(
this.$refs.qrCodeImg,
text,
this.options,
function (err) {
if (err) throw err;
console.log('success!');
}
);
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
# 选项
# 二维码选项
version
类型:Number
指定二维码的版本。如不设置,将自动选择合适版本。
errorCorrectionLevel
类型:String
默认值:M
纠错级别。
可能的值为low, medium, quartile, high
或L, M, Q, H
。
maskPattern
类型:Number
用于屏蔽符号的屏蔽图案。
可能的值为0
, 1
, 2
, 3
, 4
, 5
, 6
, 7
。
如果未指定,将自动选择合适的值。
toSJISFunc
类型:Function
辅助函数在内部用于将 kanji 转换为其 Shift JIS 值。 如果您需要支持汉字模式,请提供此功能。
# 渲染器选项
margin
类型:Number
默认值:4
二维码留白边距
scale
类型:Number
默认值:4
比例因子。值1
表示每个模块 1px(黑点)。
width
类型:Number
默认值:false
仅与终端渲染器相关。输出更小的二维码。
small
类型:Boolean
强制输出图像的特定宽度。
如果宽度太小而无法包含 qr 符号,则此选项将被忽略。
优先于scale
.
color.dark
类型:String
默认值:#000000ff
深色模块的颜色。值必须是十六进制格式 (RGBA)。
注意:深色应始终比color.light
颜色深。
color.light
类型:String
默认值:#ffffffff
浅色模块的颜色。值必须是十六进制格式 (RGBA)。
# 进阶用法
# 在二维码中间绘制logo
使用canvas
的drawImage()
方法可以在二维码中间绘制logo:
async createCode(){
const canvas = this.$refs.qrCodeImg;
const ctx = canvas.getContext("2d");
let logoImg = new Image();
let logoWidth = 0;
const { data } = await createQrCode(something...);
this.$qrcode.toCanvas(
canvas,
data.imgUrl,
this.options,
function (err) {
if (err) throw err;
_this.showQrCode = true;
}
);
logoImg.src = data.logoUrl;
logoWidth = data.width / 5; // 让logo宽度等比例的显示在中间
/**
logo 居中位置的计算逻辑为:
x轴:二维码code宽的一半再减去logo宽度的一半
(只取二维码的中间点的话,logo会默认左上角对齐中间点,
所以还需要取logo中心点,让二者中心点对齐才可居中)
y轴同理
**/
logoImg.onload = () => {
ctx.drawImage(
logoImg,
data.width / 2 - logoWidth / 2,
data.width / 2 - logoWidth / 2,
logoWidth,
logoWidth
);
};
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
最终效果:
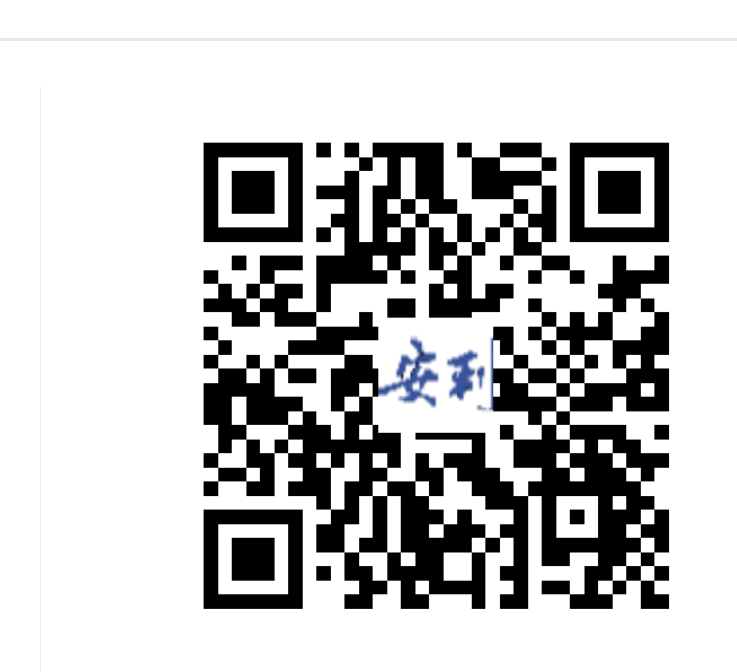